In this part of the series we are going to cover some miscellaneous(less common) as well as advance topics, which will definitely going to help you to go through a good standard interview.
Click here for Part 1
Click here for Part 2
Click here for Part 3
Miscellaneous Questions 1- Dynamic analysis
Q1) How malware disable task manager or control panel?
Answer:
Malware's can disable system utilities like task manager by modifying the security policy settings of the operating system. These policies are stored in registry at location HKEY_CURRENT_USER\Software\Microsoft\Windows\CurrentVersion\Policies\System
or HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Policies\System
(for all users) .
You can change the value of a particular key using following windows shell command:
REG add HKCU\Software\Microsoft\Windows\CurrentVersion\Policies\System /v DisableTaskMgr /t REG_DWORD /d /0 /f
Q2) What Event viewer does and how to view events log?
Answer:
Microsoft event viewer display your windows system logs about the recent activities in your system. It can give you good amount of information about the changes that a malicious program has recently done in a system. These changes include failure in authentication, disabling antivirus etc.
To view the events follow these steps:
- Click Start, point to Programs, point to Administrative Tools, and then click Event Viewer.
- In the console tree, right-click the appropriate log file, and then click Properties.
- Click the General tab.
You can read more about this here: https://support.microsoft.com/en-in/help/302542/how-to-diagnose-system-problems-with-event-viewer-in-microsoft-windows.
A good series to learn event log forensics: https://medium.com/@lucideus/introduction-to-event-log-analysis-part-1-windows-forensics-manual-2018-b936a1a35d8a
Miscellaneous Questions 2- Driver/C/C++ Development/Reversing
Q1) What are virtual function in C++?
Answer:
If you have reversed any c++ program/malware then you must have come across something called as virtual functions. If a function is defined in both parent class as well as derived class with derived class function override the code of parent class function, then that function will be called virtual function. Learn more about this here.
Example:
class base {
public:
virtual void print()
{
cout << "print base class" << endl;
}
void show()
{
cout << "show base class" << endl;
}
};
class derived : public base {
public:
void print()
{
cout << "print derived class" << endl;
}
void show()
{
cout << "show derived class" << endl;
}
};
In the above code print()
is the virtual function which have different meaning for both classes.
Q2) What are vtable in C++?
Answer:
For every class that contains virtual functions, the compiler constructs a virtual table, a.k.a vtable. The vtable contains an entry for each virtual function accessible by the class and stores a pointer to its definition. Entries in the vtable can point to either functions declared in the class itself, or virtual functions inherited from a base class. Read more here.
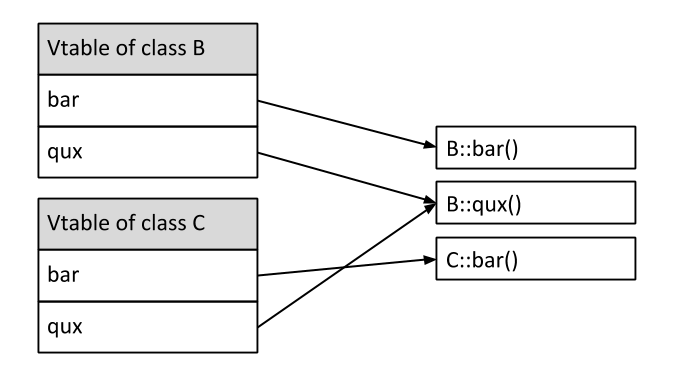
Q3) Different between reversing a C and C++ code.
Answer:
Interviewer probably want to know what you usually find weird while reversing a c++ program. There can be many different assembly behaviors of c++ program like:
- You must need to be aware that structs and objects will looks like array access while looking at assembly.
- If you find a same function, called twice with same parameter it may be the code of object creation. etc.
Q4) How to identify local and global variable in a function by looking at its assembly?
Variant: How local and global variable are different in assembly code?
Answer:
Mostly global variable are allocated or declared in .data
or .rdata
sections of the program. So, they are called directly by their address. For ex: mov eax, 0x80903030
.
Local variable are allocated in stack and usually called using offset of ebp
or esp
. For ex: mov eax, [esp-10]
.
Q5) What is DllMain in windows environment and how many times it runs when you load a dll from a program?
Answer:
DllMain is highly used by malware to run their malicious code when a legitimate program load the malicious dll's.
It is a function that by default run when a dll is loaded. Windows has provided this functionality for initialization and pre declaration related stuff. Its also a placeholder for the library-defined function.
When you start/end a program or a thread, then the dllMain is called. So, by default it will execute 4 times for a process.
Miscellaneous Questions 3- Windows Internals/architecture
Q1) What are kernel objects or Kobjects?
Answer:
There are various objects(structures) part of Windows kernel that windows use for various purposes like process scheduling, I/O management. A kernel object is a virtual placeholder (data structure) for a resource that contains information about it. Everything on a computer will have an associated kernel object like every file, every process, every port, etc. Few important objects to remember and dig deep on are:
- EPROCESS
- KPCR
- KINTERRUPT
- IRP
You can read more about these in one of my article: http://www.nixhacker.com/understanding-windows-dkom-direct-kernel-object-manipulation-attacks-eprocess/.
Q2) How process communicate with each other in windows?
Answer:
There can be many ways in which inter process communication (IPC) can happen in windows but you need to remember the ones that are mostly used by malware's or are widely used ones.
COM object - The Microsoft Component Object Model (COM) is a platform-independent, distributed, object-oriented system for creating binary software components that can interact.
In COM object access to an object's data is achieved exclusively through one or more sets of related functions. These function sets are called interfaces, and the functions of an interface are called methods.
Pipes - There are two types of pipes for two-way communication: anonymous pipes and named pipes. Anonymous pipes enable related processes to transfer information to each other. Typically, an anonymous pipe is used for redirecting the standard input or output of a child process so that it can exchange data with its parent process.
Named pipes are used to transfer data between processes that are not related processes and between processes on different computers. Typically, a named-pipe server process creates a named pipe with a well-known name or a name that is to be communicated to its clients. A simple usecase for this is remote kernel debugging. Read more here.
RPC - RPC is a function-level interface, with support for automatic data conversion and for communications with other operating systems. Using RPC, you can create high-performance, tightly coupled distributed applications.
Messaging Queues - The system passes input to a window procedure in the form of a message. Messages are generated by both the system and applications. The system generates a message at each input event—for example, when the user types, moves the mouse, or clicks a control such as a scroll bar
Q3) How mutex and critical section differ in windows?
Answer:
In short explaination, mutex require kernel interaction(kernel level permission) to work while critical section doesn't require that.
Mutexes can be shared between processes, but always result in a system call to the kernel which has some overhead.
Critical sections can only be used within one process, but have the advantage that they only switch to kernel mode in the case of contention - Uncontended acquires, which should be the common case, are incredibly fast. In the case of contention, they enter the kernel to wait on some synchronization primitive (like an event or semaphore).
Intermediate/Advance topics
Advance Questions 1- Dynamic analysis
Q1) What you can look in PE header to identify if it's malware or not?
Answer:
PE header gives few crucial details about the executable that can help you to identify if a sample is malicious or not. Before going into those details it is worth to remember a PE file can have totally normal header like other legitimate process but can be malicious. So, its not necessary that the header is definitely going to give you any required details. Now, lets look into the details.
- Having non common sections (like
.upx
) can give a hint that the executable is packed hence can be malware. .rsrc
data directory can be a good place to look for anomalies. Usually, legitimate process have icons in.rsrc
but malware may not have them but can have scripts, databases etc embedded inside them.- looking at entropy of each section, you can decide if the program is obstructed or not. High entropy(>7) usually implies obstructed section.
- Malware can have obstructed imports or no imports at all, which can give a fair hint that the program is trying to hide its imports.
- Looking at the imports you can guess what are the activities the program is trying to do. For example if you find a normal text editor have imports for functions like
winsock2
,regopen
andCryptoAPI
then it can be a fishy program(altough those imports can be used by a total legitimate process also.
You can check this research paper to understand little more about this.
Q2) How to know if a process in memory dump is a malware or rootkit using volatility? (Memory forensics)
Answer:
In short: You can dump the process memory using memdump
plugin and start analyzing them.
Detailed: You can use following methods to identify a malicious process in memory dump:
- Use
pslist
to list the processes with their pids. - Use
connscan
to view the network connection done by a process. - Use
dlllist
module to list the dll's and dump it usingdlldump
. - You can use
malfind
plugin to find the malicious process. This can be help to detect process injection.
There are more plugins that you can use like handles
plugin to find much more information about the process. While analyzing if you find any anomalies in the data then you can conclude that the process may be malicious. Read more here.
Advance Questions 2- Assembly Language
Q2) X64 calling convention.
Answer:
The question is not at all advanced but mostly ignored in generic interviews. Although as a researcher it is must to have good understanding of x64 assembly and calling convention. Compare to x86 calling conventions(cdecl and stdcall), 64 bit system calling convention differs in these areas majorly:
- Arguments are passed using registers rather then pushing into stack. For windows the registers used for arguments from left to right is
RCX
,RDX
,R8
,R9
etc. For linux the pattern isRDI
,RSI
,RDX
,RCX
etc. - Cleaning of stack is done by caller just like
cdecl
calling convention. - Stack aligned in 16 bytes boundary.
You can read more about x64 calling convention(specific to windows) here.
That's it for this part. In the next part we will look at more advance questions and most important linux malware analysis related stuff.